One of my tasks for the project I am working on was to create a function that allows users to send an invitation link to their candidates. So, for this functionality, I had to implement “send email” on our web application. In order to achieve the task, I had researched. I found there are two packages: nodemailer and emailjs. Long story short, I decided to use nodemalier because it is free and there is no limit on sending emails (but emailjs, you have to pay to use it or if you are willing to use the free version, it is limited to 200 emails per month).
What is the nodemailer?
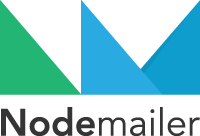
Nodemailer is a module for Node.js applications to allow sending email.
How to implement sending email function with nodemailer?
You might be able to find many video lectures online, but most videos are dealing the function when if it is in the same server(or Port). However, if you working on react, as you already know, the front-end and back-end are running on the different server ports. In my case, the front-end is running on port:3000 and the server is running on port:7000.
So I have to pass JSON data from 3000 to 7000. So, without middleware, you cannot achieve that.
In this case, Axios can be a good solution since you can send the data to the server port with Axios. And, here is the code I implemented:
Write Axios code on your front-end function where you want to evoke sending email
axios({ //call axios method: "POST",
//set url with your server port
url:"http://localhost:7000/send",
data: { //build a data form
name: emailSubject, //Send send
email: emailAddress,
messageHtml: emailContext
}
}).then((response)=>{ // Waiting response
if (response.data.msg === 'success'){ //display result
alert("Email sent, awesome!");
}else if(response.data.msg === 'fail'){
alert(`Oops, something went wrong with`)
}
})
Server side code:
const express = require('express'); //load packages
const nodemailer = require('nodemailer');
app.use(express.json());
app.post('/send', (req, res)=>{ //catch post send
console.log(req.body)
const transporter = nodemailer.createTransport({
service: 'gmail',
auth:{
user: "from email address",
pass: "password"
}
})
const mailOptions = {
//build mail option
from: "quizbanana467@gmail.com",
to: req.body.email, //use data from front-end
subject: `${req.body.name}`,
text: req.body.message
}
//send email
transporter.sendMail(mailOptions, (error, info) =>{
//send feedback to front-end
if(error){
console.log(error);
res.json({msg: 'fail'});
}else{
console.log("good")
res.json({msg: 'success'});
}
})
})
Based on the email service you are using, there would be some limitations so please refer to the official nodemailer site.
//references
mailtrap.io/blog/react-send-mail