While file structures and the like are incredibly entertaining, at some point in the development of a text based game were probably going to have to parse some text. But before I dive into developing a natural language parser (next week hopefully?) why don’t we work on some behind the scenes stuff that enables the user to change the game world in a meaningful way?
I’m going to be breaking down how I build up relationships between objects. It doesn’t sound like much but its essential to making a game environment that persists. Our goal will be to build two rooms, a player, and move between them. Pretty basic movement but to run you must walk, and to walk you must stand!
Class Room
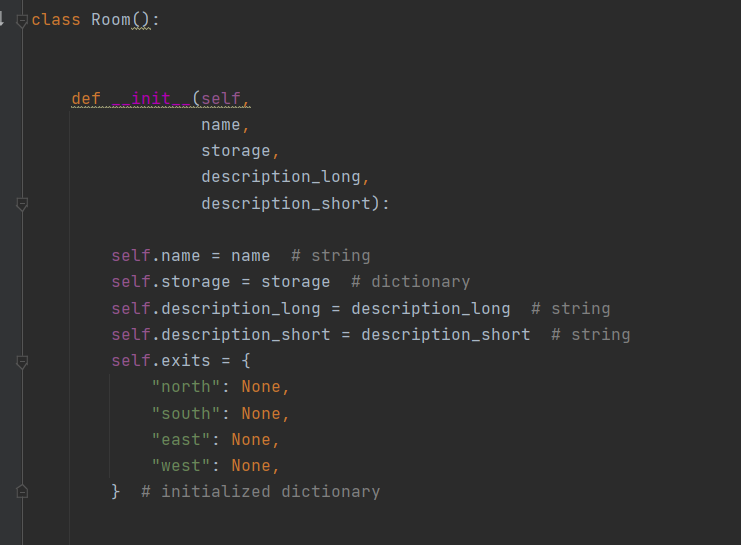
You’ve probably seen a class like this, its mostly string attributes for its name and a couple descriptions. Then we have the .exits dictionary where we will spend most of our attention.
Class Player
We need something to “move” between rooms right? so lets make a quick player.
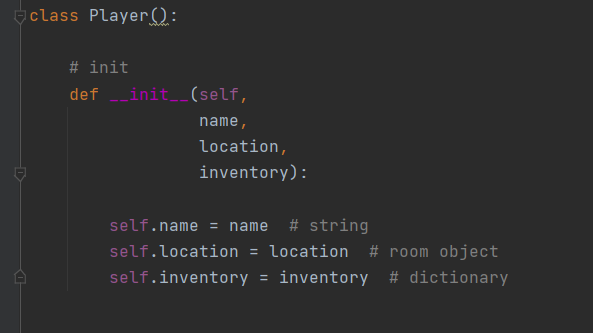
There nice and short, we gave him a name, an inventory that we will ignore for now, and most importantly a location.
The location that we passed here is noted as being an object. This is important because we want that object to reference later. If we need to ever tell the player where they care we can just call location.name and it will give us the name string we stored on the Room object when we created it. I like to think of this as one way association. The player knows that they are in the room but the room doesn’t know that the player is there.
The last part we need to cover before building a room relationship is making instances of room objects. Lets make a Den and a Library. Oh and an instance of our player.
Initialize Rooms/Player
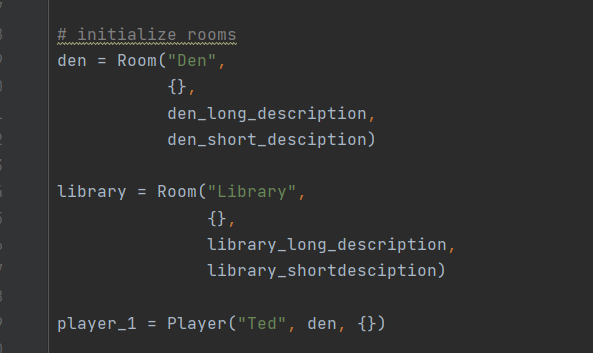
For the Rooms we just pass each a name string, a blank dictionary and a couple of variables that represent longer strings (keeps the code clean)
Then we make a guy, lets call him Ted. Ted starts in the den so we will pass him that object as his location. Ted has nothing in his inventory. Poor Ted.
Building Relationships
Ok we have two rooms, and even a player who knows he is in a room. Now we need a hallway between the Den and the Library. Lets put it on the west side of the Den and the south side of the Library because the hall curves for some reason.
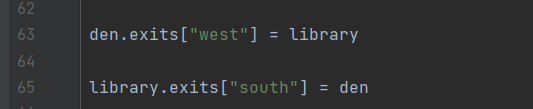
We do this by accessing the .exits library in each room and going to our desired direction key. Then we set that direction to the other room. That gives us a reference within each room object to the other room. I like to think of this as a two way relationship as opposed to our one way earlier. The rooms both know that they are connected! Don’t think about it too much!
First steps
Now is the moment of truth our player got tired of the Den and he wants to go Read a book so he inputs a command that calls room_mov() one of his methods with the string “west” because he remembers the library is over there.
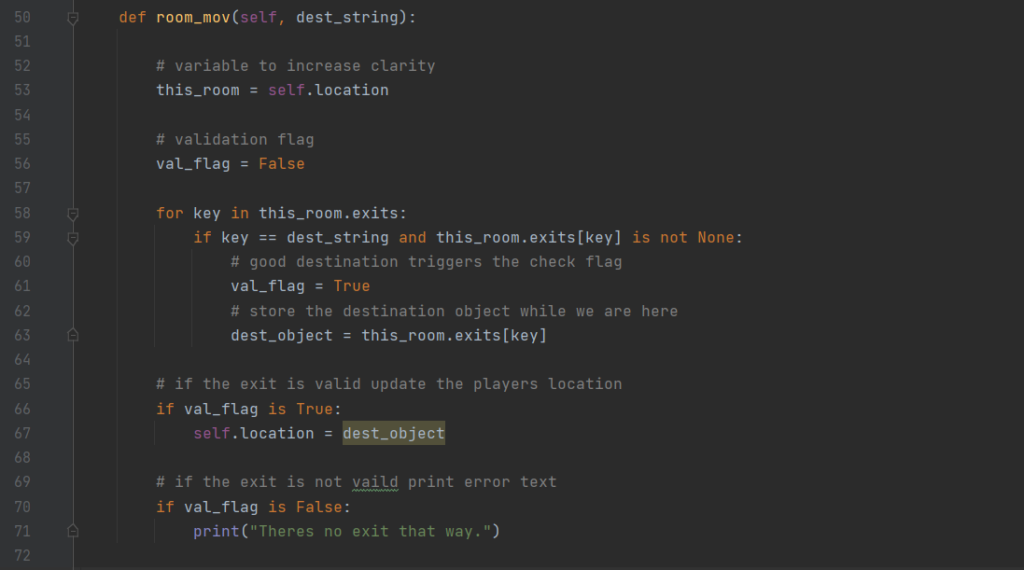
His method room_mov() looks a little dense but its really just checking to see if west if each direction has a brick wall or a hallway.
First we set a bool and rename self.locaation too just this_room because its a little easier
the for loop on line 58 iterates through keys for all the possible exits from the den (north south east and west)
We check two things for validity on line 59, wither the key matches the key string the user gave us, and wither that key has an association attached to it.
If both of those are true then its a valid destination! Yay! we set our bool to true to indicated this on line 61
For convenience sake we also grab the validated object that matches the key string on line 63.
THAT WAS A LOT OF VALIDATION
but it was worth it because we get to update our players location on line 67 and he finally gets to Read the latest copy Of Fantasy and Science Fiction magazine that he has been waiting all month for.
At the end we can check for non existing associations (brick walls) by printing out a string that lets the player know they are still in the same room. Wrong way Ted.