In my current project I am at some point going to need to save data to a file and load the data back in the next time they are going to use the program. With Python there are a few ways to do this but the most common seems to be using JSON. The problem I found in much of the existing material available after a quick google is that an individual tutorial might tell you a piece of the information you need but many of them gloss over crucial steps in order to tell you five different ways to turn your data into a json dictionary. Great information but less that practical. I’m going to show you a way that works start to finish. Is it the best? Well it works so lets start there.
Remember to import json before you start coding.
Moving a dictionary into a JSON file
In order to save anything into a JSON file you need to covert it into the proper format. This is where json.dumps() function comes in. You can see below that I declared and example dictionary and then converted the dictionary by passing it to json.dumps()
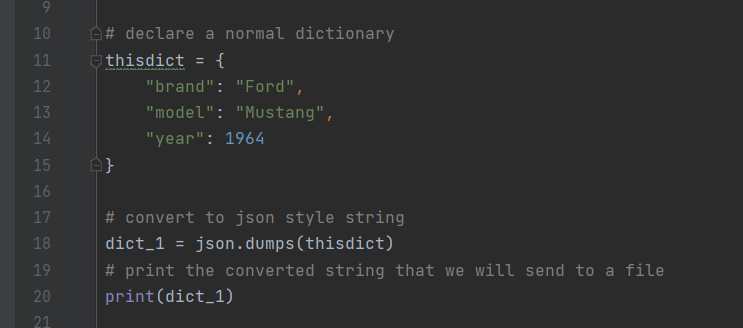
If you print it out at this point you will see that the file has been changed into the JSON format.

The next step is to take this formatted information and save it to a JSON file. To do this we can use with open(). When we pass the function these parameters with open will either make or open a file called dictionary_save.json note that this can be whatever file name as long as it ends in .json. We will also pass the “w” argument because we are writing to the file. Note that when we refer to the file in this section of the code have designated it thisdict_file. Very handy we want to do anything with that file we just went through all the trouble of creating. The very last thing here is to use json.dump() and pass it the formatted data we saved to dict_1 above and the file we want to send it to. Good thing we have that reference to our file.
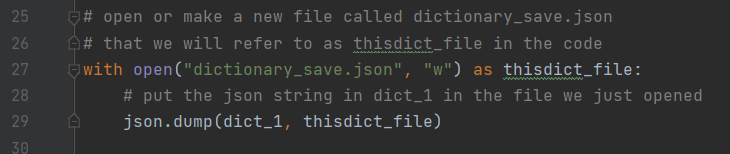
If you run the program right now you aren’t going to see much difference. but if you open where ever your folder for the program it or you can see it in your IDE you might notice an addition.
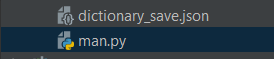
Its the file we just created! If you open it you can see that your data is stored in there.

This is how it looks in the python editor.
Moving a dictionary out of a JSON file
Cool now you information is saved now you need to know how to access it when you need it. This section has two parts, you have to retrieve the json data and then turn it back into something you can use.
You can use with open() and pass it the file name you are trying to access and the ‘r’ argument and it will let you read the file. We also tell it we are going to be referring to the file as thisdict_save for this section of code.
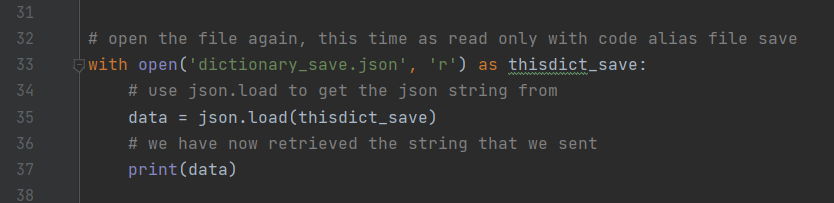
We also have a chance to use another JSON function here, json.load(). This will grab the data from our file and save it to our cleverly named variable.
If you print the data variable the output should look something like this

In other words exactly the same thing we sent in.
I have to say that I am a little put out that some guides decided to stop here in their explanations. After all you got the formatted information back but you can’t do anything with it. Useless.
That’s where this little one liner comes in.

json.loads() turns JSON formatted data back into a dictionary that you can reference normally. There is a subtle difference in the prints but if you have double quotes around you dictionary when you print, it is likely still in JSON format as in the first line below.

Single quotes on your dictionary mean that you have it back to a workable Python format.
CONGRADULATONS!!
You just stored a dictionary in a JSON file and got it back to a working Python dictionary. Small victories add up to something larger.
Check out my GitHub for the full code I used for this explanation.