One of my goals in my current project, making a text based adventure game, is to create a method of saving the state of the game and loading it later. Last week I went in depth on a method of saving dictionaries but that falls a little short when it comes to a larger project. What would be really useful is the ability to store the attributes of a given object and retrieve them later. Then ideally if I created a new instance of an object when the game opens, say representing a room, I could simply write the saved attributes over the ones it is initiated with. That way if a player “dropped” and item in the room it would be preserved in one of the attributes. This could also be used to save states of features in the room, or other changes that the player makes. Additionally it allows the room class that the object is created from to inherit all of the methods that might be tied to it, eg. get exits, get description, that kind of thing. Here’s what I came up with.
same as last time remember to import json before following along.
Basic Setup
We will need an example class to work with, so we will initialize a basic car class and give it a few attributes as well as a method. Honk probably would have been a better method but I’ve ben watching too much Knight Rider lately so our car can talk.
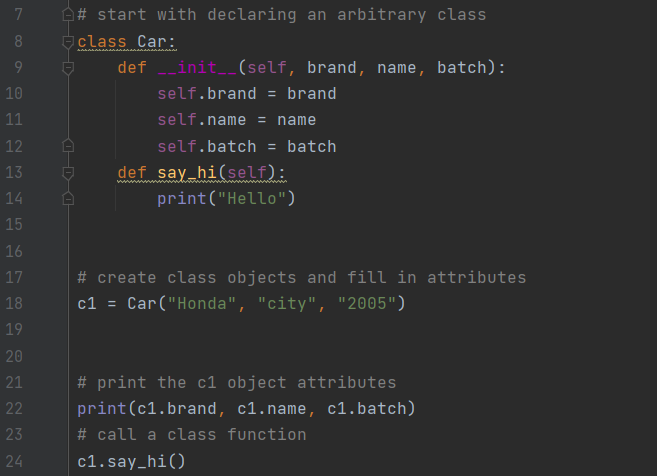
Its always good to throw in a couple test prints just to make sure the basic stuff is working. Your current output should look something like this

Boom we have a talking Honda city. Its no Pontiac Firebird but lets try to save it anyway.
Save the attributes
While JSON is an incredible tool it seems that it mostly likes saving dictionaries. There are more complicated ways to store a whole object but I found this method to suit my purposes the best. We are going to save our attributes as a dictionary so we can restore them to a new instance of the object at a later point. We can to this with a method very similar to the dictionary saving I covered in my last post.
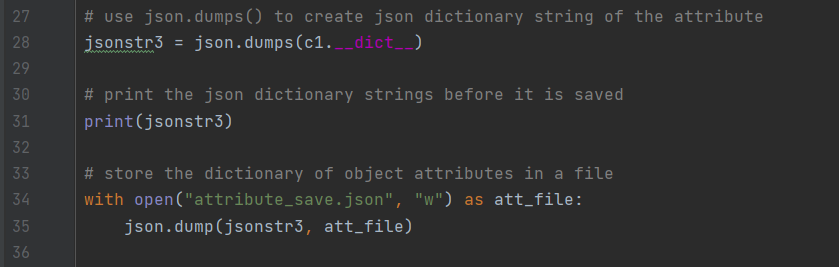
The first think we need to do is convert to JSON format so we use json.dumps() to dump a dictionary of our objects attributes. Very handy. Throw in a test print to makes sure the string looks right and then make a new to a JSON file using the same with open() command I introduced in my last post. Finally we can use json.dump() to send the formatted string to the file that we just created. As with last week your output shoudnlt change much but you will notice a new JSON file named attributes_save in the same folder as your main python folder.

Now that we have a dictionary of our object attributes saved pretend we are starting a new instance of our game. We ultimately want to make a new car with the same attributes as our first so lets start by grabbing them from the saved JSON dictionary.
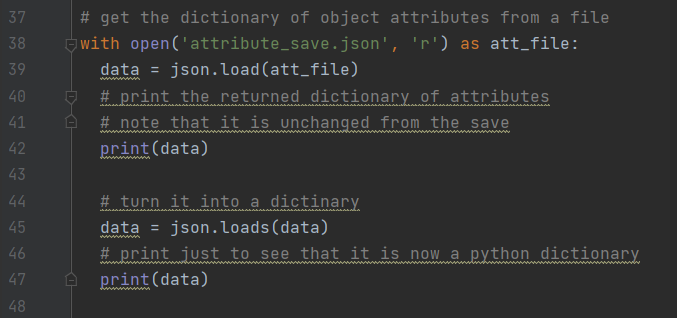
We can use with open() and the ‘r’ attribute to read the JSON file. Then we save the new data by running json.load() with the file alias and assigning it to the varible “data” on line 39. We then use json.loads() to make it a python dictionary. This pretty much the same process we used to get JSON data in the last post. This time however, after we confirm that the data is good with a couple test prints we are going to do something different.
Make a new object with the old attributes
This bit is quick but essential, don’t blink or you’ll miss it.
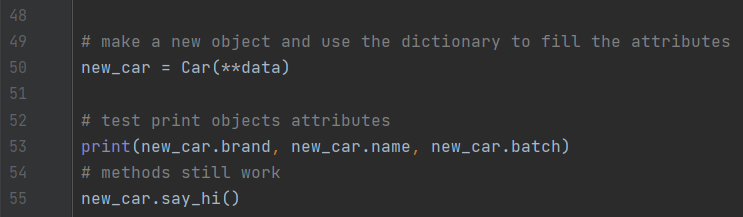
Ok so what happend there.
Lets start with the first bit, we created a new car object. When we do that it should have the initial attributes all cars start with, but those are effectively junk values when we want our cool Honda City back. When we make the call to make a Car object we pass in **and the dictionary of data that we retrieved as the attribute values for our new car. Thus we have created an object that is essentially exactly the same as the one we saved. Since new_car was created as a Car object it also has access to all of the same methods as the original. The only draw back to this approach is that you have to know ahead of time what the attribute fields of your object are but it works for the purposes of saving.
you should at this point be able to get the old values from the new_car object! Well done!
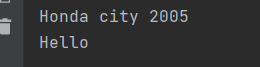
Here’s a link to my get hub with the complete code for reference.
https://github.com/samuelh223/Json_objects_dictionary/blob/main/main