This week I dabbled with creating the counter label and some functionality for the previously made add/remove buttons, but once again I spent the bulk of my time working with buttons.
With much of the base UI complete, I needed to really start getting into the nitty gritty of getting tiles placed where the designer pressed on the screen, but I had one big question–how on earth do I do that? Posing my query to another engineer over coffee, they posited that one way I could accomplish this would be by turning the screen into a grid of buttons. My wheels immediately began spinning and I was keen on bringing this idea to fruition.
Coding alongside my mentor, we started by creating a single button that used our tile texture so that the button on the screen looked like one of our blank Mahjong tiles. Having created together a single tile button that appeared on the screen at the specified point {{x,y}, z}, my mentor challenged me to extrapolate that into a function that would return a unique pointer of a TileButton and to then use that function to create a grid.
As per usual, bumps were encountered along the way, but eventually I had written a function named CreateButton that took in the rect of the board and a TileId, and returned a TileButton that could be pushed onto the Views vector. On the screen it looks a lil something like this:
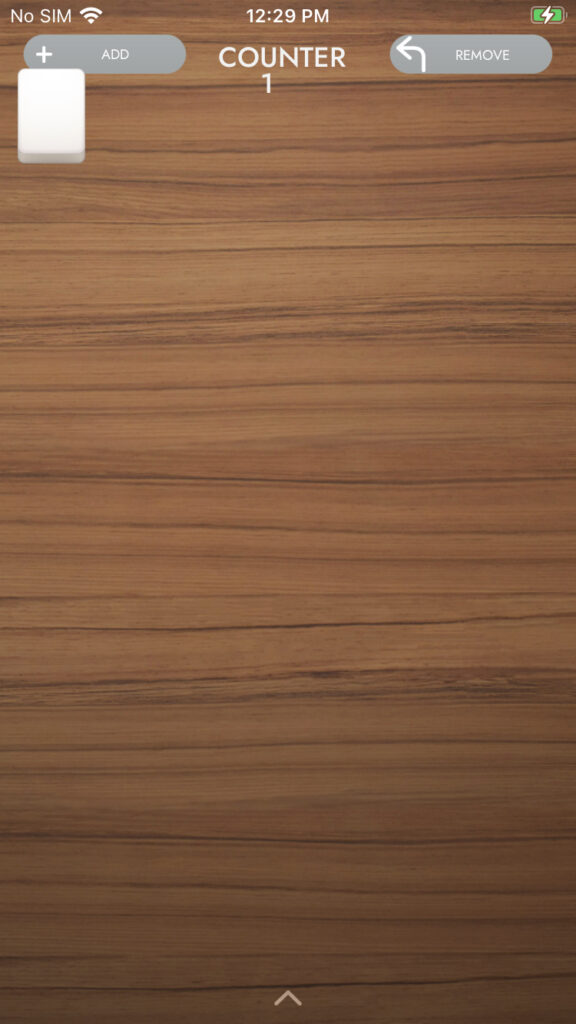
Next I needed to create a grid of tile buttons, which would be a vector of unique pointers of TileButton. Filling the vector was pretty easy as I was able to push a new TileButton onto the button vector using the CreateButton() function I’d written, like this:
buttonVector.push_back(CreateButton(rect, tileId));
Getting the buttonVector into the views vector to display on the screen proved to be an embarrassing challenge for me. As I’d previously done with adding other elements to the views vector, I added a line in my GetActiveSubviews function:
views.push_back(&*buttonVector);
The compiler was not pleased and gave me an error I didn’t understand to which I turned to Google and Stack Overflow for assistance. This got me nowhere.
After a lot of Googling and searching through the codebase for a similar situation, I reached out to my project mentor via Slack to talk through the issue. I described that I was trying to add a vector<unique_ptr<T>> (where T is a subclass of View) to a vector<View*>. I was consistently getting hung up on how to “add” one vector to another, as though it had to be something particular with one vector being filled with unique pointers causing .push_back(vector) to not work and that I needed to use type casting or perhaps a different function for adding the vector.
But as you surely know, vector doesn’t have a function that allows you to insert another vector into it. To add a vector to another vector, you need to implement a for loop that moves each item in the vector to the other vector (which, if vector did have a function to add a vector to itself, that’s how it would have to be implemented).
This “Aha!” moment left me with a lot of mixed feelings. I was pleased to be moving forward with pushing my vector of buttons onto the views vector by adding each button to the views vector, relieved that my code compiled, and satisfied that the grid of buttons had been created. But I was also feeling a bit of shame that I didn’t clue into this elementary concept sooner and without so much hand holding. I know that a vector is essentially just a list and that if you want to put each item from one list into another you are essentially looping through to do the job, but for some reason this knowledge was just out of reach at the time of application.
This likely won’t be the last time some coding knowledge that I’ve previously learned doesn’t come to the forefront of my mind when it needs to, and I’ll continue to work on being kind to myself when it occurs; in the end, the goal is still to learn and grow, and this week I certainly learned something important about vectors.
The latest iteration of the Board Editor with a grid of “ghost” tiles for designers to select and add to their board design:
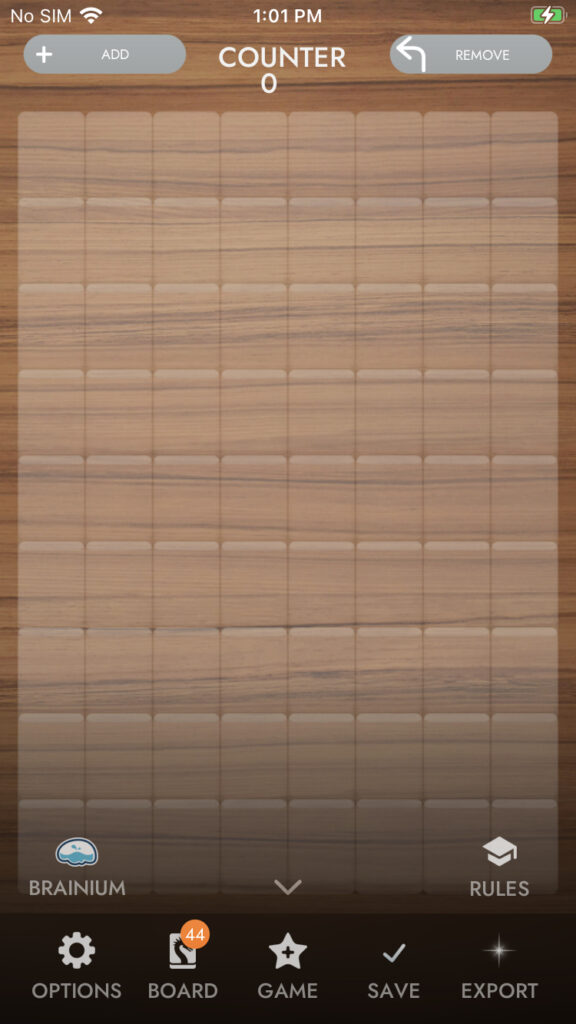