I like turtles.
Sorry, I couldn’t resist bringing that meme back. As you can tell with my voice in the background, they are just too darn cute.
But wait! Before you go, if you found this page, I bet you’re wondering what this blog is and why you’re here.
This blog is for students and professors wanting to know how to survive as a college student. It is also for them to see the heartbreaking and exhilarating process of failing and succeeding when wrestling with software projects for the first time.
In my opinion, there is so much more to do than use software tools. So if you are interested in:
- Understanding why they work
- Exploring why they are built the way they are
- Brainstorming why and how they are useful for different types of communities
Then you are in the right place!
How I Got Here
My name is Kaitlyn Hornbuckle, and I’m a computer science student at Oregon State University. My education focus is on cybersecurity1, but I also dabble in content creation, science writing, public speaking, search engine optimization (SEO) techniques, and course development for various companies. Oh, and I love cats.
Below is a map detailing the steps I took, five years after enrolling in my first out-of-state university. The first stop is my original major.
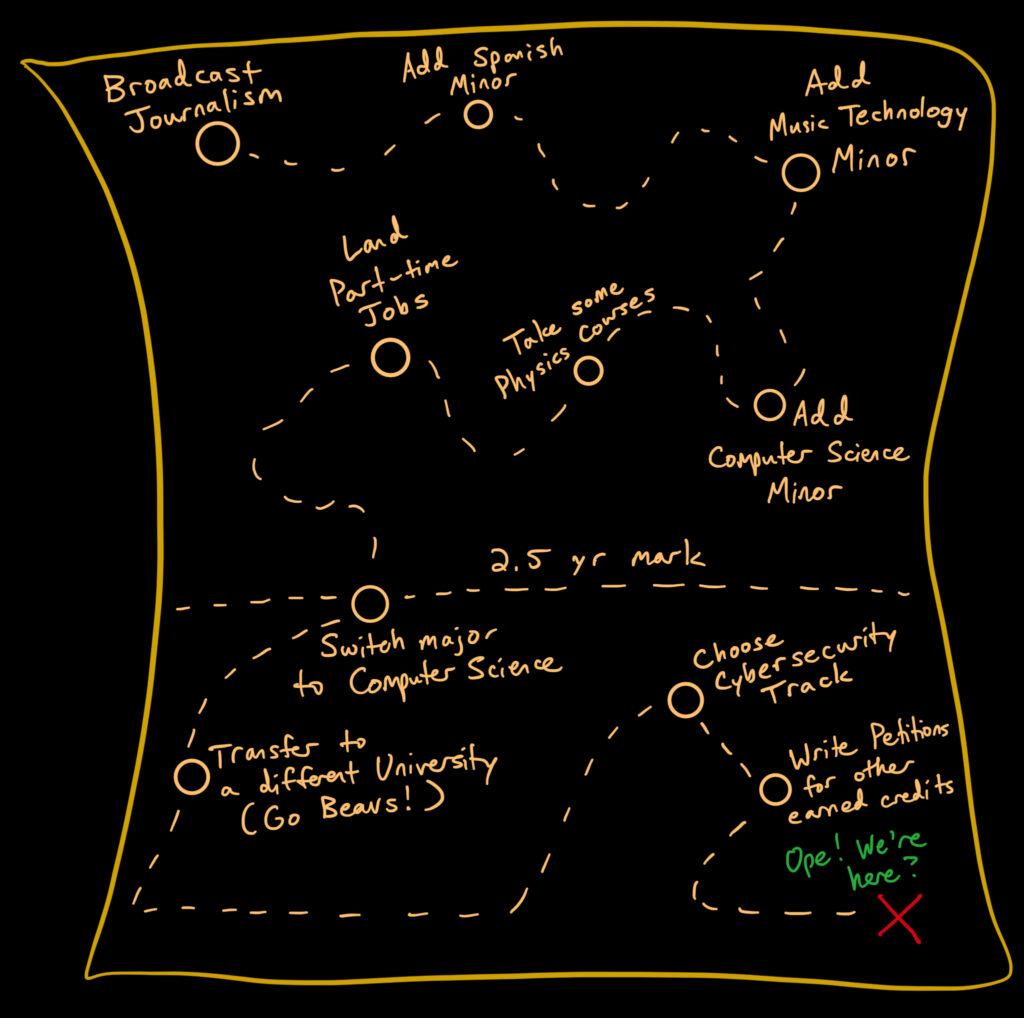
As you can see, my journey was not a straight line. But it did lead me to a myriad of adventures in the field, including but not limited to video editing, writing online tutorials and science articles, teaching science, technology, engineering, and math topics to K-12 students in camps and workshops, and building a Discord bot that combats hate speech.
How I Started with Computers and Software
After I recorded my first video in the early 2000s using bubble effects from laptop camera software, I fell in love with everything related to technology.
In elementary school, I enjoyed spending my time exploring everything I could on the Internet, and then spread the word with my friends. I dove into Webkinz2, Moshi Monsters3, Club Penguin4, Spineworld5, CoolMathGames6, and found a website where I wrote a love letter to Justin Bieber (to this day, I wonder if my email address got sold for that one, but oh well). As word spread, it wasn’t long before the school’s network blocked almost everything I loved. But I also enjoyed running around outside in the fresh air, so I couldn’t complain.
When I got a little older, I defended Minecraft villages with my friends and screamed from everything — axes, creepers, zombies, empty caves — you name it.
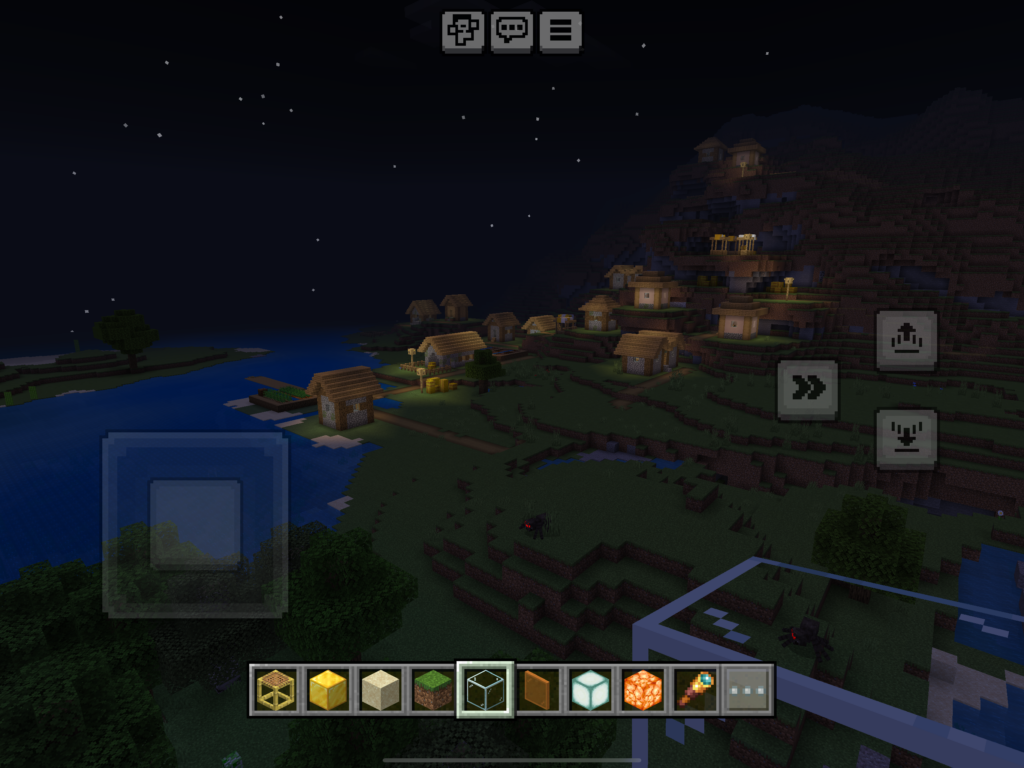
In high school, I accidentally did Batman’s job (he was the IT guy at my high school) with the printer in my journalism class. I sat in front of video editors, audio editors, 3D animation tools, text editors, photo editors, search engines, graphical user interfaces on operating systems that constantly updated themselves, spreadsheets, video games, various Integrated Development Environments (IDEs) and emails.
But I don’t think I officially started with computers and software until I realized using them can help people. That was when my passion of using tech tools transformed into a raging passion for strategizing which technical projects and soft skills are useful and beneficial for communities.
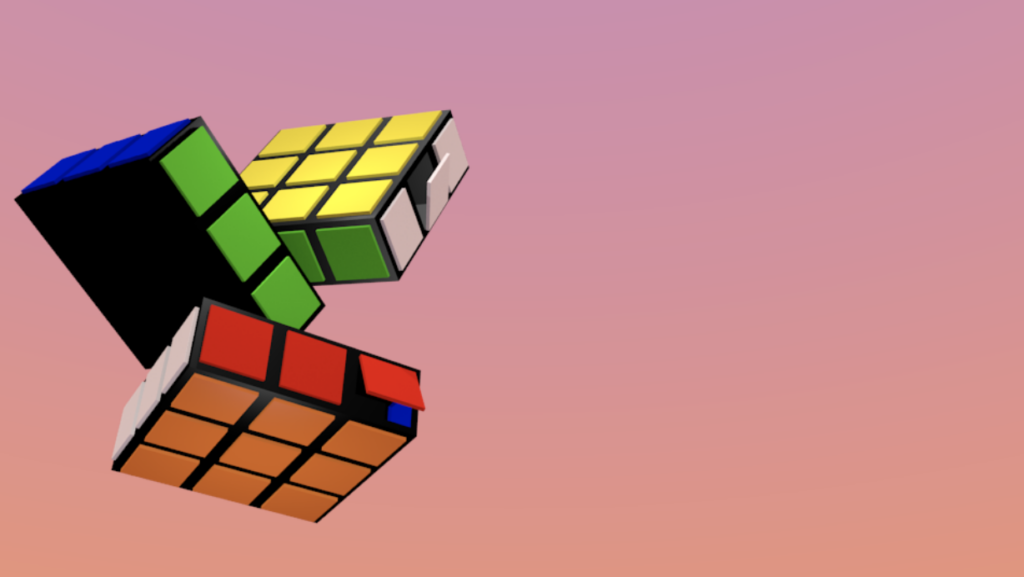
Why I Jumped from One Technology to the Other
Once I figured out that I could use technology to help people, I dove deep in education technology. I could list off a bunch of methods and tools I learned along the way, but that’s for a different article.
However, there are three technologies from other industries that I want to highlight, as they provide new learning opportunities not only for students, but for product development and security training as well.
Hugging Face
Yes, their mascot is a hugging emoji. Despite the friendly name, the company provides a lot of machine learning documentation with thousands of open source models, datasets, and demo apps. It’s a relatively big collaboration platform that could be helpful for building any AI products in the future, both small and large.
Kali Linux
As someone who is new and wants to learn advanced penetration testing and security auditing techniques, this Linux distribution is the perfect toolbox. Personally, I’m curious about finding a way to use its customized kernel to test the website security of a custom site. The tools are best used ethically, and their tool documentation is a great starting place to explore.
Python Anywhere
For a long time, I struggled to find ways to practice using cloud software because I’m a student with a low budget. I also wanted to find a way to teach Python without requiring students to download anything, and that’s where I found this platform.
As someone who learned about ‘the cloud’ and what the purpose of a console was late in the game, I wish I found PythonAnywhere earlier. The platform allows you to access a console, create web apps, store code, and run 24/7 automated scripts. Some features are free to get started, but I made the $5/month option work to run small always-on tasks. In my case, it was for running and designing a Discord bot.
So with a little bit of exploration with these artificial intelligence, machine learning, cybersecurity, and cloud tools, I’m excited to see what types of projects I write about next and learn how to measure their impact.
Conclusion
I hope you enjoy the rest of the blog. Before you leave for your own adventure, don’t forget to enjoy the silly things in life — like how my cat doesn’t let a kitchen chair get in the way of attacking straws:
And if you want to stay updated on my latest career developments, feel free to check out my LinkedIn.
Happy coding!
- Cybersecurity Option at Oregon State University. https://engineering.oregonstate.edu/Academics/Degrees/computer-science/computer-science-option-cybersecurity ↩︎
- Webkinz. https://www.webkinz.com/ ↩︎
- Moshi Monsters Wikipedia. https://en.wikipedia.org/wiki/Moshi_Monsters ↩︎
- Club Penguin Wikipedia. https://en.wikipedia.org/wiki/Club_Penguin ↩︎
- Spineworld Fandom Wiki. https://spineworld.fandom.com/wiki/Spineworld ↩︎
- Coolmath Games. https://www.coolmathgames.com/ ↩︎
- Minecraft. https://www.minecraft.net/en-us/about-minecraft ↩︎
- Blender. https://www.blender.org/ ↩︎