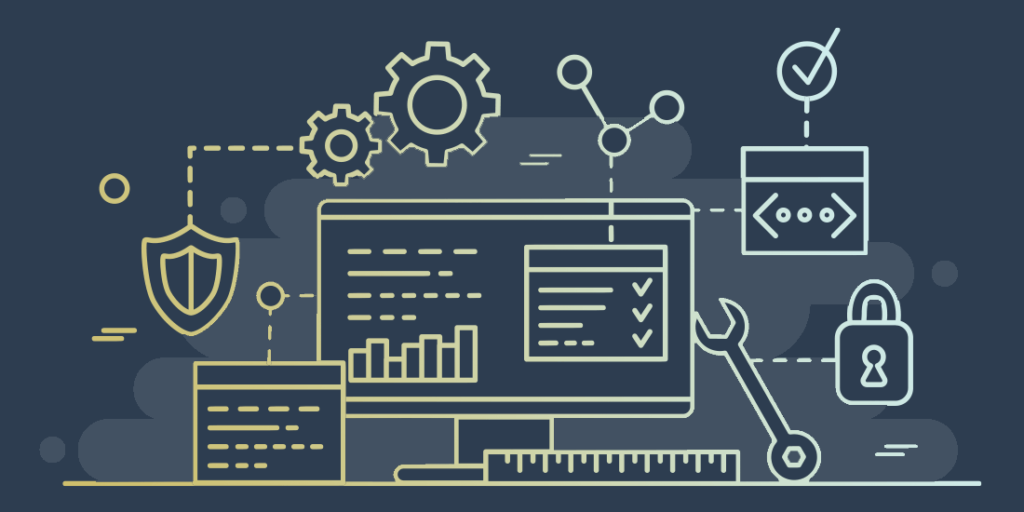
Authentication is the act of proving an assertion, such as the identity of a computer system user.
Wikipedia
This week, I’m working on authentication for our capstone project. My first experience with auth involved securing API routes using Auth0 during my Cloud Applications Development course. In this blog post, I’d like to discuss how Auth0 acts like a middleman to authenticate users, how it secures user data, and why developers should consider outsourcing their app security to a company like Auth0.
Auth0 is a platform that handles the complicated process of authenticating users. Administrators configure Auth0 to handle user logins by choosing the type of login to allow i.e. Google, Apple, username/password, etc. Then admins simply provide Auth0 with URL redirects for successful logins and for unsuccessful logins. Auth0 essentially becomes a middleman and the app flow looks like this: a user attempts to login on your website and is sent to an Auth0 login form. Once authenticated, Auth0 sends them back to your website using the specified redirect URL. Generally a cookie is stored locally to confirm to your app that they are authenticated. Under the hood, Auth0 secures sensitive data like usernames and passwords in AWS by salting and hashing data using bcrypt. What is salting and hashing anyway?
Salting hashes sounds like one of the steps of a hash browns recipe, but in cryptography, the expression refers to adding random data to the input of a hash function to guarantee a unique output, the hash, even when the inputs are the same.
Auth0
Let’s look at a real life example of what happens when we hash passwords without a salt. Looking at the 2012 LinkedIn Hash Dump Analysis, 1.13 million people used the password “123456”. If a bad actor decrypts this one hash, he or she now has a password that works for over one million accounts. Hence, salting helps to eliminate duplicate hashes, thereby increasing the security of all your user accounts.
In addition to salting and hashing, Auth0 encrypts this data at rest in the MongoDB database and in motion (during the back and forth requests between Auth0 and the database). It uses a cyrptographic protocol called Transport Layer Security (TLS) with at least 128 bit encryption. This simply means that the length of the key to encrypt the data is at least 128 bits and generally up to 256 bits. While this sounds complicated, there are libraries to help developers with encryption, so why don’t we all just build our own home grown authentication solutions?
One big downside to individually handling authentication is responsibility. The developer is now responsible for all the security and maintenance of the authentication system. This means that every time security best practices change around password hashing or storing user data, the solution must evolve. Depending on the size of your company or developer team, this could be very doable or a major headache.
For our capstone project, we are building an application for a small business that needs authentication for its employees. Luckily, Auth0 has a free tier when you have less than 7,000 users, which is perfect for a small business. Outsourcing security to the professionals frees up our developer team to spend more time solving business problems, ensures tighter software security, and overall is the optimal solution for our use case.