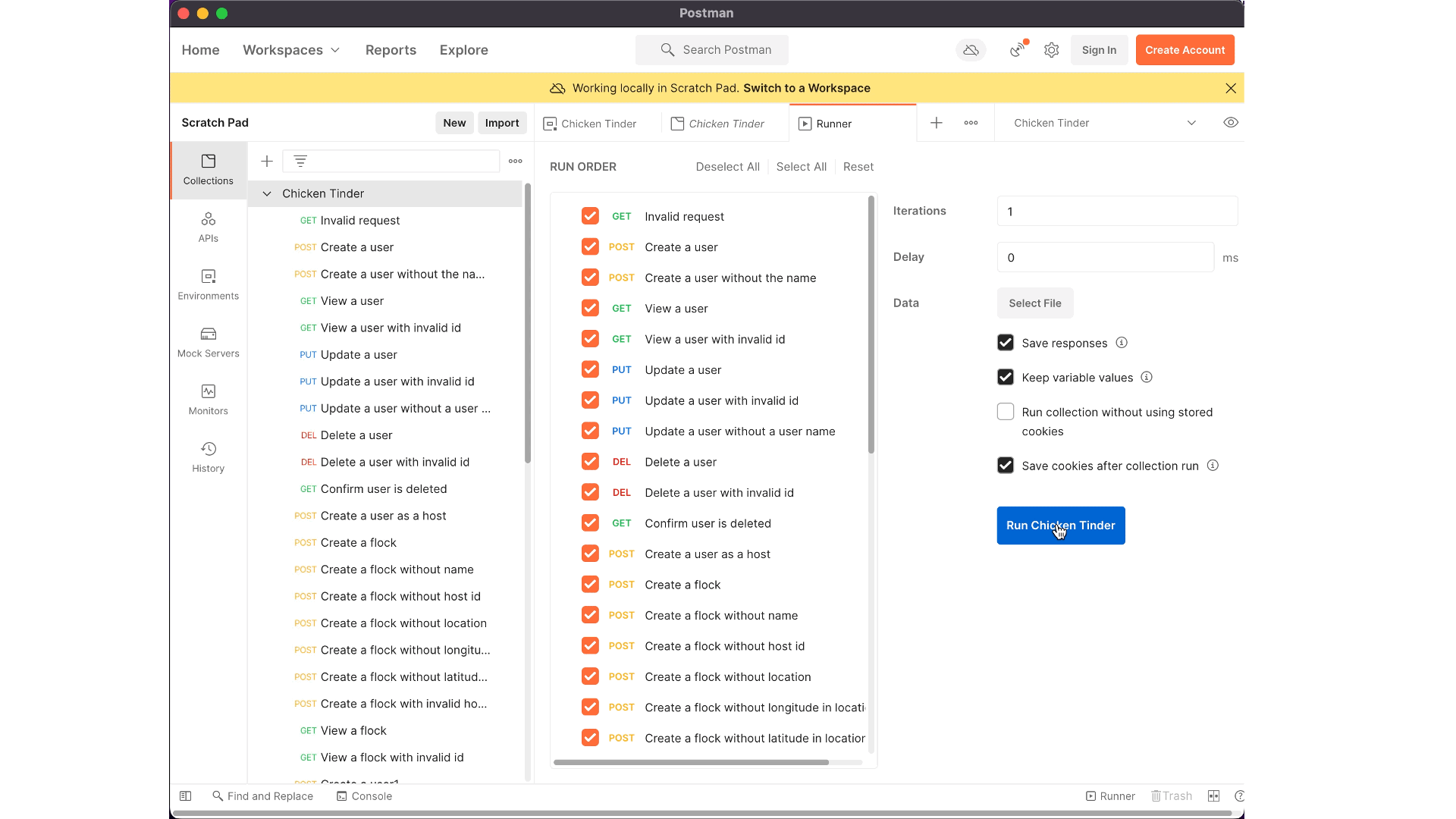
While creating the backend api for Project Chicken Tinder, I sometimes lose focus on the requirement of the project. There are times I don’t know which piece of code to start first. And there are times I missed steps and have to spend extra time traceback my errors and false assumptions. While this is part of learning to build larger and more complex projects, I have found Test Drive Development really keeps me focus and reduce the complexity of the problem.
The app idea is to have a beautiful and functional frontend built by React Native and make http calls to the backend via RESTful API. While I was brainstorming this design, my approach was to create two different entities to be stored in Google Cloud Platform (GCP), flock (like a flock of chickens) and users.
Users
{
user_id: user1_id,
user_name: "Charlie
}
We will only be storing the users’ name in this entity as we want to make this application as simple as possible. While we could have save this under flock, I do want to keep it as a separate entity in case we want to store more user data and preferences in the future.
Flocks
{
flock_id: flock1_id,
flock_name: "Capstone Team's Lunch",
host: xxx,
location: {
longitude: 40.4406,
latitude: -79.9959
},
restaurant_votes: {
restaurant1_id: 0,
restaurant2_id: 2,
restaurant3_id: 7,
...
},
user_votes: {
user_1: 10,
user_2: 7,
user_3: 5,
...
},
restaurant: [
{ ...restaurant1_data },
{ ...restaurant1_data },
{ ...restaurant1_data },
...
]
}
Other than the obvious information about the flock (i.e. flock_id, flock_name, host, location), we will be storing each user’s number of available votes and each restaurant’s number of votes in the flock entities. As a result, we can increment each restaurant’s vote count and decrement each user’s available vote count every time a vote request is made. I also made the decision to request the nearby restaurants based on the flock’s coordinates in the backend and saved under the flock. There are three major reasons for this decisions:
- Yelp Fusion API comes with a daily limit of 5000 requests. If every client make the request in the frontend, then this limit will be deplete quickly. If I only make this request in the backend and save to the database, I will only use 1 API request for every flock, no matter the flock has 2 people or 20 people.
- Yelp Fusion API also requires an API token to accompany every request and as a developer I certainly would not want this key expose to the public.
- There are many unknowns under the hood of Yelp Fusion API. Different set of restaurants can be pull from the same coordinates depending on the time of the day, changes in ratings, or some other mysterious factors. In order to ensure all users within a flock are voting on the same set of restaurants, saving this data in another database and relay this information seems to be the only choice.
So what does all of these have to do with Test Drive Development?
Creating a project from scratch gives me flexibilities and opportunity to be creative. However, the lack of well define requirements also contribute to my feeling of being lost and unsure about my decisions. May be I don’t know how all the pieces fit together in code but I have a good idea of how people actually want to use this application. Therefore, I adopted the Test-Driven Development approach partway through my development progress as I was feeling lost and un-focus. I used the testing suite in Postman to automate my testing. I creates tests for my application as if I am the user and my collection of tests tells a story of how users create account and flock, join other existing flocks, and votes on their favorite place to eat. As I was creating these tests, I also think about how invalid or in-compliant requests can be made to my API so I can catch as many edges cases as possible. Time flys by and many test cases were made to ensure the robustness of the API backend. Whenever I did some refactoring or minor redesign of the code, I can re-run the test suite to make sure I did not break any existing functional codes. Last but not least, there was also the satisfaction of seeing my code passes 136 tests in seconds. I really enjoy using TDD for this project and I will look into how to implement this test suite with CI/CD and explore other testing frameworks.