Ever heard of CORS? For those who are not familiar with web development, CORS may be a new concept.
It stands for “Cross-Origin Resource Sharing” and there are great online resources that go over the concept such on the MDN web docs or a quick overview provided on the wikipedia page. Here’s a brief description taken from the wikipedia article on CORS:
Cross-origin resource sharing (CORS) is a mechanism that allows restricted resources on a web page to be requested from another domain outside the domain from which the first resource was served.
– Cross-origin resource sharing on Wikipedia
So What Exactly Is It?
Say you created a full-stack web application and you want to test it out locally. You’ve selected two different ports to run the frontend and backend:
- http://localhost:3000/ for frontend
- http://localhost:8080/ for backend
Frontend wants to fetch some data that’s stored in a backend server that’s hosting a database, which is currently running on the address http://localhost:8080/ so frontend decides to send a GET
request to the backend with http://localhost:3000/ as the origin point.
Depending on how the backend is setup, one of the three responses will be returned:
- Send back the requested data along with
Access-Control-Allow-Origin
(ACAO) header in its response, letting the requester (frontend) know that request from that particular origin (http://localhost:3000/) is allowed.- It may look like:
Access-Control-Allow-Origin: http://localhost:3000/
- It may look like:
- Send back the requested data along with ACAO header in its response, letting the requester know that requests from all domains are allowed with a wildcard (
*
).- It may look like:
Access-Control-Allow-Origin: *
- It may look like:
- An error if the server does not allow a cross-origin request
- If you have the Web Developer Tool on your browser open, you may see it in the console
It’s a way to prevent unauthorized (and unintended) HTTP requests from being made; same-origin security policy is also in place for majority of the major browsers used today in order to protect the users of these browsers from ending up in a wrong place. Browsers don’t want users to end up like Dorothy telling Toto “I’ve a feeling we’re not in Kansas anymore” like in The Wizard of Oz.
My First Hand Experience
As a naive, newbie developer that only had few experiences of coding my own personal website prior to attending OSU, when I took CS290 (Web Development) and CS361 (Software Development I) and was tasked to develop a full-stack web app during these classes, I was destined to encounter and figure out how to handle CORS.
Spoiler alert: I did.
Even after reaching a point where I’m currently developing a capstone project with my teammate, CORS has still made a comeback and managed to block our path. Since my teammate and myself have looked into this for a good few hours one afternoon/evening, I’ll be sharing how we resolved it (and later found more material on it).
The Error

So what exactly is going on here? For quick context, we’ve decided to create a React-based frontend with a backend server that’s run on node.js and express for our capstone project (called Kaizen Manager).
When one of our team members pushed the change to the Github for our project and set up the Pull Request, I decided to test the frontend to backend connection locally, but encountered the above error when we thought we appropriately configured each end of the web app.
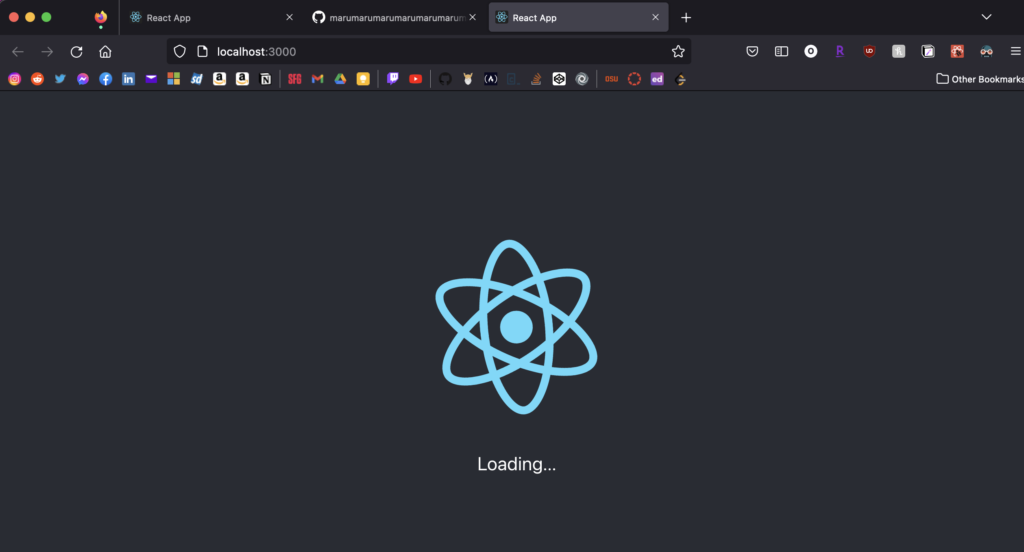
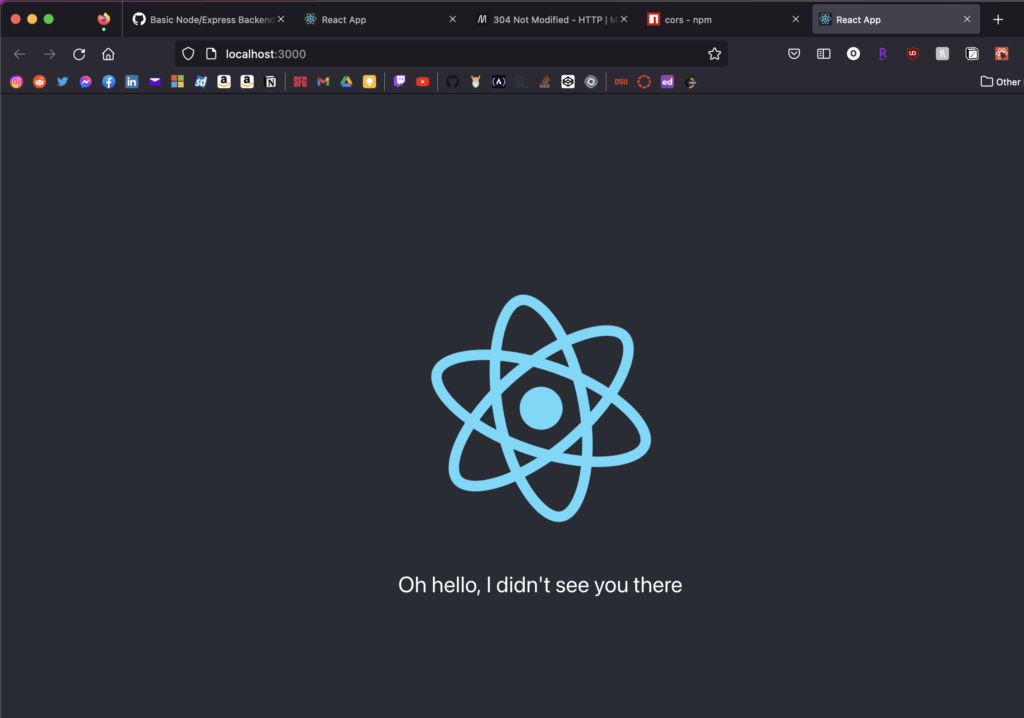
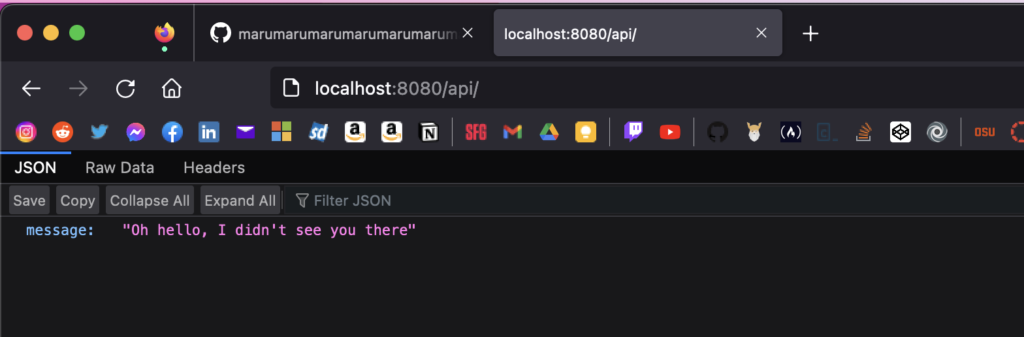
As we can see in the first image, we’re encountering CORS issue. The backend server on localhost:8080/
isn’t accepting the request getting sent by frontend over on localhost:3000/
. What should we do?
The Solutions
We’ve come across few solutions over the course of the CORS battle, and I’ll be sharing two of the solutions we’ve found and those that we tried with our project.
One way is using the cors package, the other way is to simply define a proxy.
cors package
npm is a package manager for Javascript programming language and you can find useful packages created by other developers for you to use in your code. cors is one of such handy packages and by installing it to your project, it enables CORS.
CORS is a node.js package for providing a Connect/Express middleware that can be used to enable CORS with various options.
– Readme of the cors Module
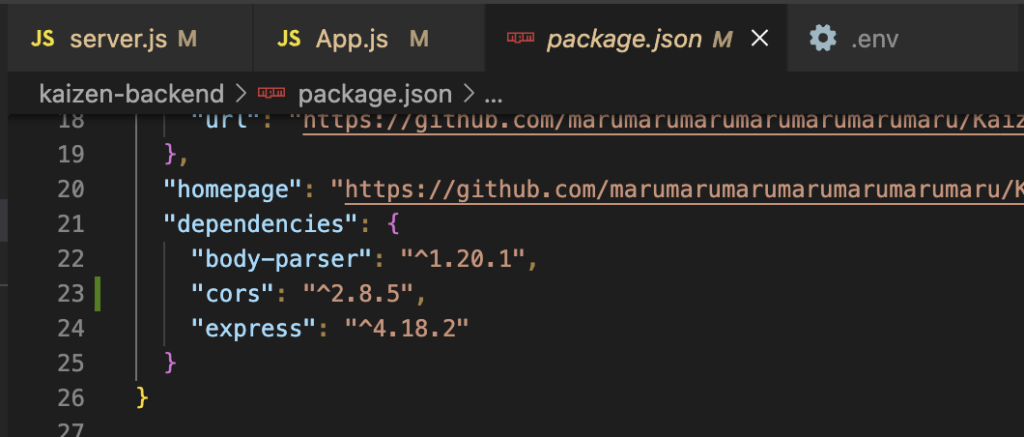
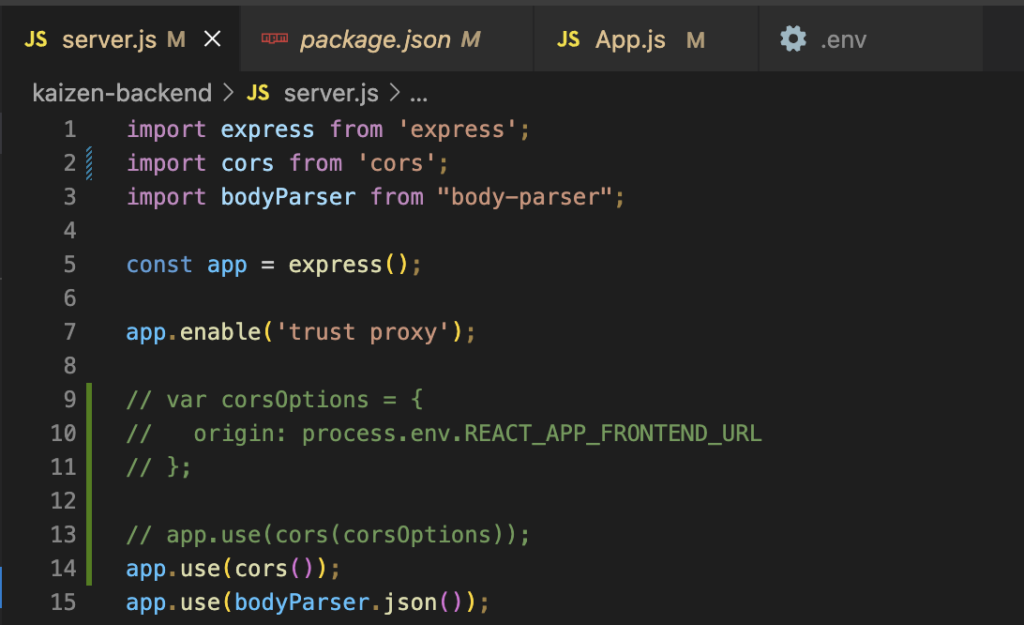
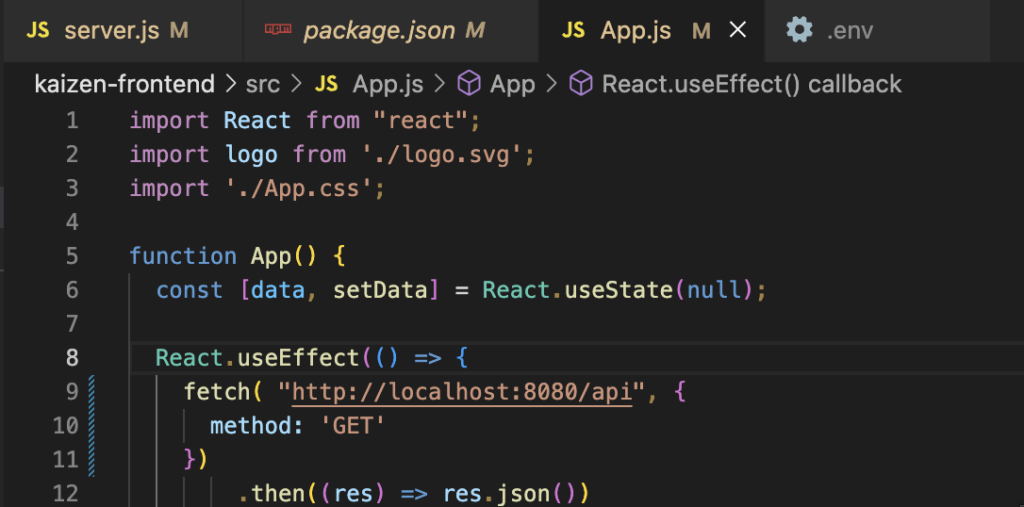
Then, the cors
module will handle the rest. It’ll allow the request coming from any origin (based on the wildcard *
) or a specified origin (the commented out code example from above) and send the data back, allowing data to flow from backend to frontend.
Set the proxy
This was discovered after learning about the cors module. React actually has a very handy way of handling CORS issues by defining proxy
in the package.json
file. See details here.
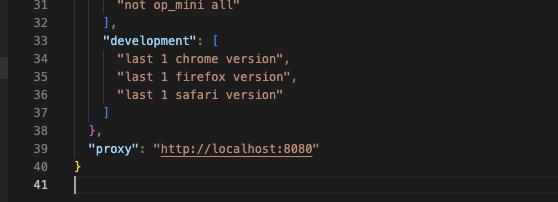
My teammate and I were a little dumbfounded by this simple solution; however, in the official documentation it is noted that this is only works in development (when we run the app using npm start
).
In fact, in the same documentation from create-react-app.dev website, there’s a link on how to enable CORS on ExpressJS. This is something I remember attempting to implement in my previous encounter before my capstone project, but did not work. I definitely need to try it out locally and make sure I understand what is going on.
Lesson Learned
After all these encounters with CORS-related shenanigan throughout my web dev study, I find myself realizing that I still got a lot to learn; however, I do find myself becoming better at finding potential solutions and being more comfortable discussing technical problems with my teammate, which is a great asset (skill) in itself.
In fact, writing this blog post was a great way to better understand the solutions I have found at hand, and I’m excited to continue coding on my capstone project further. So, for now, thank you for reading and,
Until next time.
– Fusako