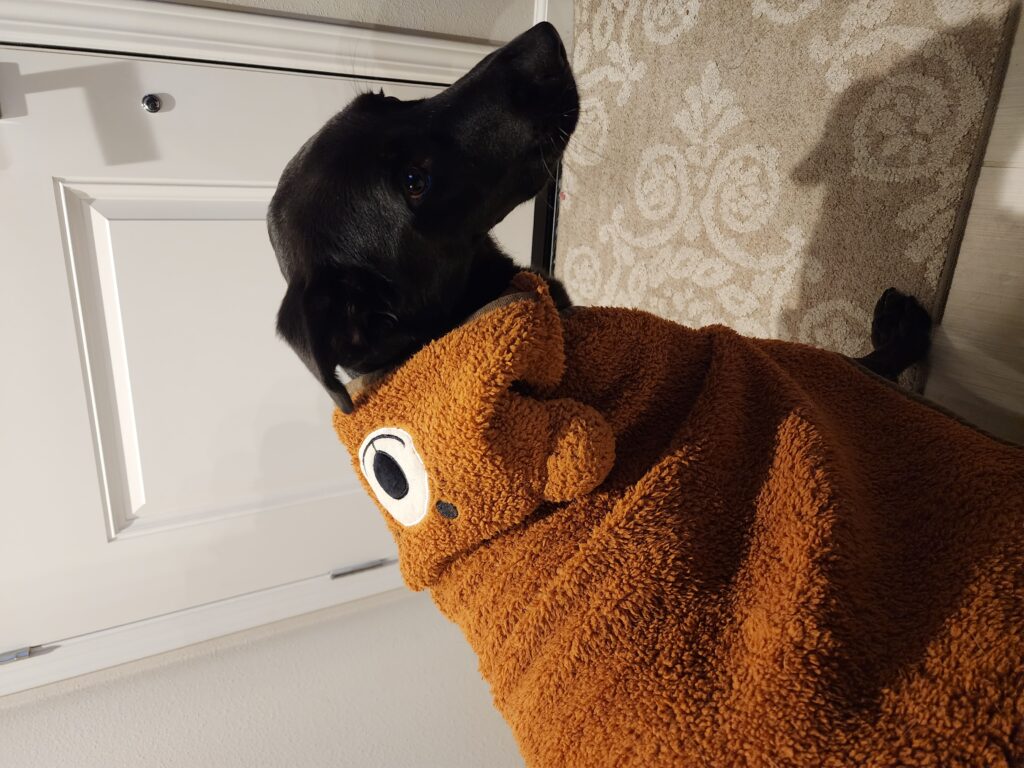
(Sorry that the title sounds depressing – I assure you that I kept it optimistic in the end.)
We are now less than 1 month away from wrapping up this term! It’s crazy to see how speedy the time passed since I got accepted into the program and begun studying at OSU from Winter 2021. It was also around the same time that I got an offer to work for Facebook’s (now Meta) security program management team, and I’ve been working at Meta as a contractor for almost 2 years now. It’s been a busy ~2 years, but I learned a lot.
I started off as one of four technical project manager/coordinators on the team, beginning my role around mid-December of 2020. I’m now a team lead of the same team that is now about 14 people, which is an impressive jump now that I think about it.
Why am I talking about my current job all of a sudden?
You may have guessed from the title, but this week – specifically, on Wednesday November 9th 2022, Meta announced that they’ll be laying off 11,000 employees (about 13%). Am I impacted? Well, fortunately I wasn’t impacted by the first wave of this layoff. At least, from what I can tell, as I haven’t received the bad news yet directly. However, Wednesday has been one gloomy day – internally, we are calling it a “Metapocalypse“.
This isn’t my first time at a company laying off employees; in fact, I was one of the people who got laid off from Amazon in the beginning of 2020 (right before the COVID-19 layoff wave that happened early in 2020) due to reorganization. I knew something was off few months prior to the bad news, when the projects I’ve been working on were getting shifted to another team in different office. The suspicion was confirmed when I got a meeting scheduled with my manager along with few other invitees – of course, it was the HR and director, looking a little sheepish and guilty, delivering the news to me (and others).
It’s been almost 3 years since that incident and that has given me a lot of time to digest, reflect, and move on from it. So, here’s few pointers and tips that I can share to someone who may be going through a layoff, or who’ve never been in a close situation but might want to mentally prepare one’s self for it, just in case:
- Don’t get too comfortable in a position, even if you love it. Make sure to update your resume time-to-time.
- Challenge yourself. Along with updating resume, maybe go through an interview once a while to see how you compete, or do some coding challenges. Keeping your skills (both technical and interview) sharp is important!
- When you get laid off – it might feel like a mountain in front of you or falling into a pit. Take time to digest, take as much break as you need/can get, and start making strides forward, big or small.
- Most importantly – be kind to yourself. Most of the time, layoff isn’t your fault.
I know I’ve got still a long way to go in my career and these pointers may not resonate to everyone, but I hope for those people who are still early in their career that may have been laid off to keep their hopes up and be kind to themselves. I’m also planning to start looking for a new role in December as well, and while I’ll be competing in a whole different market where many tech people will be looking for new opportunities – I hope that I can find the right company that would be a good fit for me.
Until next time.
– Fusako