Test-driven development (TDD) is a software development process relying on software requirements being converted to test cases before software is fully developed, and tracking all software development by repeatedly testing the software against all test cases. This is as opposed to software being developed first and test cases created later.
https://en.wikipedia.org/wiki/Test-driven_development
From a couple weeks ago, my team finally started coding for our capstone project. For those weeks, my task was to create backend API endpoints. I followed Test Driven Development (TDD) to create those endpoints as I already knew the requirements for the endpoints.
This week’s plan was to create four endpoints below for our “A Dating Application for Animal Adoption” app.
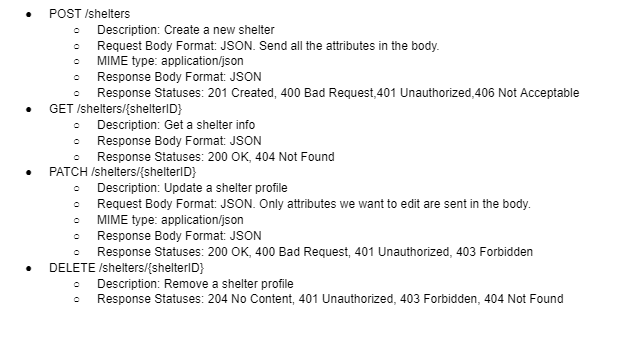
I followed below six steps to implement those endpoints.
- Write a unit test for one endpoint
- Write the bare minimumĀ of code to pass the test from step 1
- Run all the written tests
- If tests all pass, return to Step 1
- If the failing test is still failing, return to Step 3
- Evaluate and refactor the code as necessary to avoid duplicate code
- Stop development after adding “enough” tests and tests all pass
//Example Unit test by using npm supertest library
it('POST /shelters responds 415 (incorrect content-type)', () => {
return supertest(app)
.post('/shelters')
.send(
"shelterName:Shelter Portland1"
)
.set('content-type', 'text')
.expect(415, "Incorrect content-type.")
});
TDD helped to avoid duplicate code since I wrote a small amount of code at a time to pass the tests. Also, I was confident that my code worked as expected. When I refactored the code, it was also easy to verify if my changes worked as expected. On top of that, if my teammates changed something, they don’t need to write any test cases. The tests are already in the code base and it is reusable any time.
I haven’t tried TDD at work nor previous projects, but I am glad that I used it for this project. To create API endpoints, there are other useful tools such as Postman, but TDD is also very easy to use and it is highly recommended if you have a chance to try it.