Started working on the jobs/internship page this week. I had designed the mockup, so I know what it needs to look like.
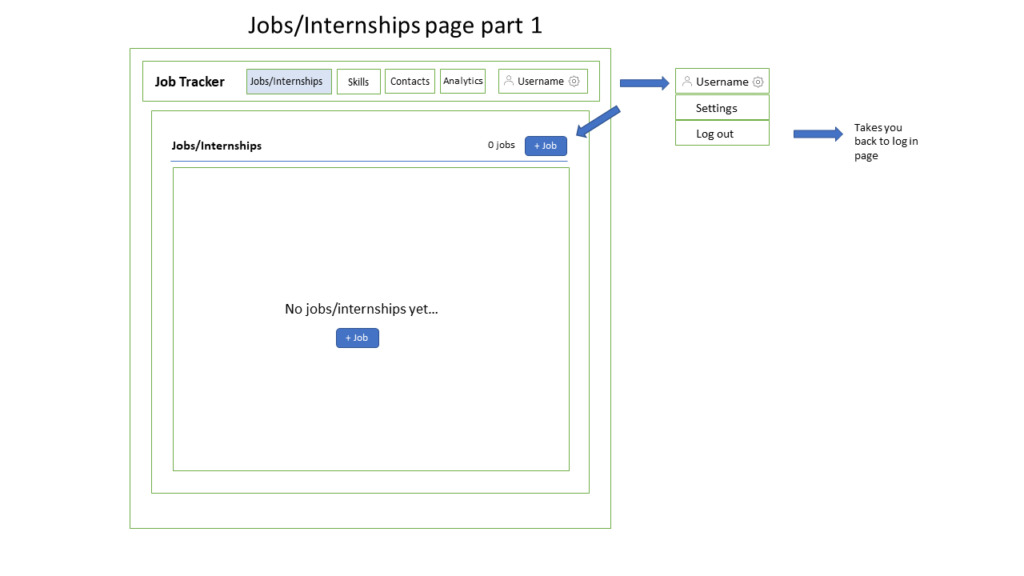
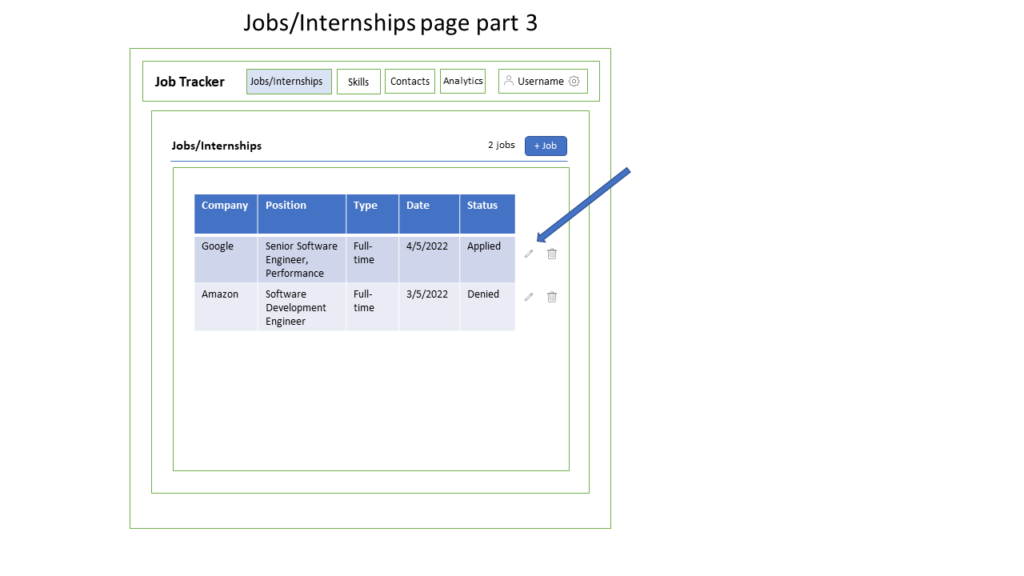
Functionality that I want:
- The button will open up a form modal
- If there are no entries, there will be text saying there are no jobs and another form modal button
- The items in the form add to the table
The database was not set up yet, so I had to use fake data. I created an Application object that has the attributes we want (company, position, type, date, status). I made a few fake applications so I can simulate data in the database. This way I did not have to hard code entries into my HTML template.
Remember, we want to keep our code DRY (‘Don’t repeat yourself’)
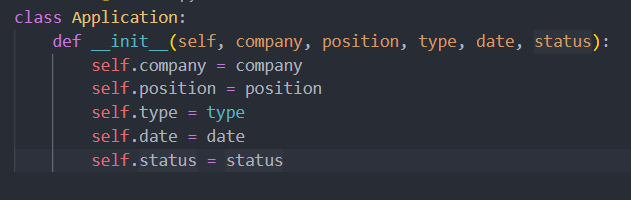
After coding for a while my template file was getting really long and cluttered. I used my form modal and button twice (1 for the top of the table and 1 if there are no jobs yet). There has to be a way to make my code cleaner, preferably modular. Having the ability to separate parts of the code that I can use elsewhere would be ideal. It took a long time and a lot of research, but I found it! Thanks to this post on StackOverflow, https://stackoverflow.com/questions/1976651/multiple-level-template-inheritance-in-jinja2
I was able to separate big chunks of code and ‘include’ them to the template I want.
After getting the layout to behave the way I expected, I started looking toward implementing the database entries. My teammate provided the documentation for firebase and then I looked into it. I added some entries to the online database and my goal was to connect and read the values. On firebase, they are called documents in a collection. So, I will refer to the entries as documents
Following this tutorial, https://cloud.google.com/community/tutorials/building-flask-api-with-cloud-firestore-and-deploying-to-cloud-run.
I was able to connect successfully and assume I was able to access the data. Here was my output.

I was stuck on figuring out how to display each of the object values for the longest time. The tutorial kept using jsonify(). I decided to try removing it and it outputted what I wanted!
Here, you can see the two entries I created:

Side tip!
I recently discovered the python function, dir(). This is very helpful because it shows what functions an object has. Here is an example output of using it

Doing this allows me to do many things with the collection object.
What helped me solve a lot of my issues this week was to walk away when I got stuck. I walked my dogs, ate a snack, and even just called it quits for the day. Stepping away I feel like my brain can reset and different ideas come to my head.