Today I got an interview at a small startup in Los Angeles. The startup works with motor controllers, and only has 11 employees (including the 2 founders). I got the interview because I knew somebody who worked there. This was my first technical interview, and it didn’t go very smoothly. Because it happened today and I still have a very fresh memory of it, I figured it would be a good opportunity to write down the experience so that I can more easily look back on it.
Take Home Project
Before I even qualified to take the technical interview, I had to create a small react project and send it to the team. The task was to create a button with the company’s logo on it. When a user clicks the button, a value (displayed below) is incremented. The tricky part was that the state had to persist despite page refreshes. I had never persisted data like this in react before, but found that react has something called localStorage which was built explicitly for this problem. It was not difficult to throw it together so I made sure it was as perfect as I could. I even added some nifty CSS animations on the button press where the logo would spin counter clockwise. Finally I had to write a test suite for the app, which was actually really fun too. They liked my implementation and offered me the opportunity for a technical interview.
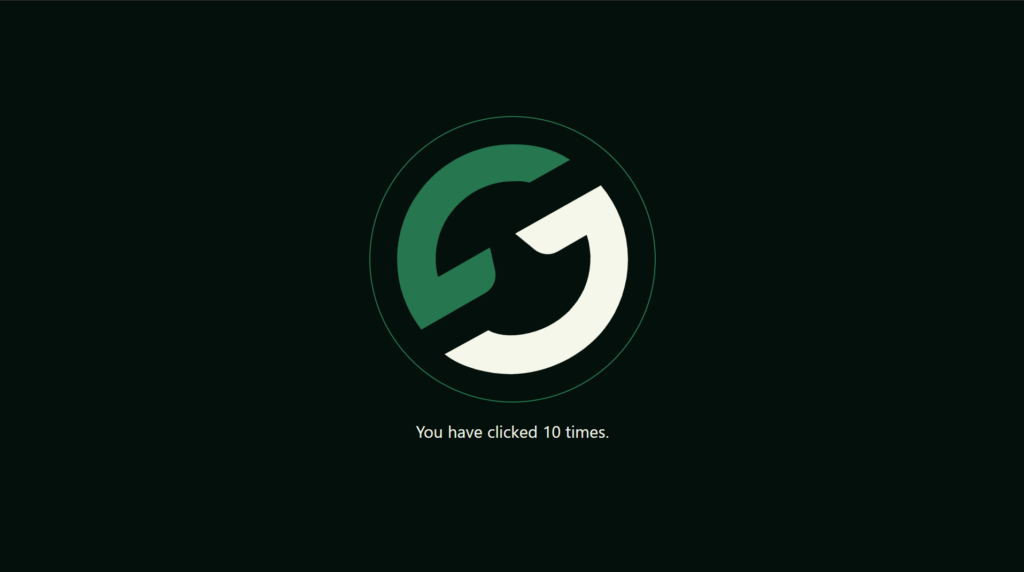
First Interview
The first programming problem came from the senior software engineer for the company. He sat me down at a provided laptop with VSCode and a powershell window open with the problem.
Problem 1
I found that this problem was probably taken from leetcode. The problem they gave me did differ slightly in that it expected a returned matrix rather than editing the given matrix.
You are given an n x n 2D matrix representing an image, rotate the image by 90 degrees (clockwise).
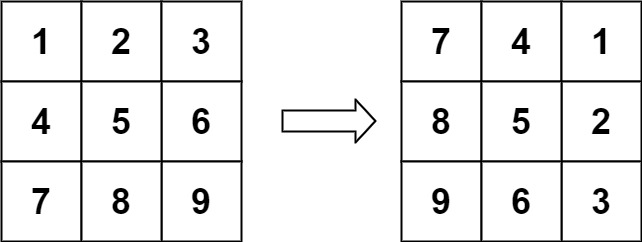
At first I thought that this required you to rotate only the outside layer, but when I looked more closely at larger matrices, I found that everything had to rotate.
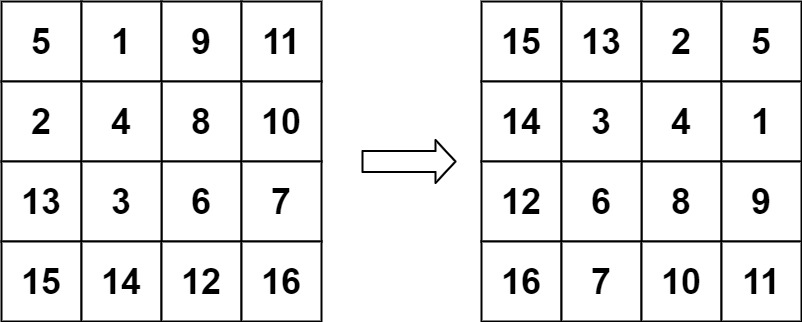
It took me quite a bit of time to figure out what approach would be best. I couldn’t really wrap my head around the problem at first. Eventually I arrived at this plan of attack:
- Have 2 for loops incrementing values i and j from 0 to the size of the array (n)
- These values represent the positions on the rotated array
- Before the j loop starts, I initialize an array called row
- As we iterate through the j loop I push values onto the row
- Values are determined by the relation of the rotated matrix’s numbers to the input matrix.
- Return the rotated matrix
I did this in typescript but I’m not very good with typescript so I’ll try to show my implementation in python
def roateMatrix(matrix):
rotated = []
n = len(matrix)
for i in range(n):
row = []
for j in range(n):
row.insert(0,matrix[j-n][i])
rotated.append(row)
return rotated
I’m a little unsure of why when doing this problem again I got reversed rows and had to append to the front. Probably just my memory about what exactly the formula for finding the right value for each spot.
I barely got this done on time, and required quite a bit of help. I think the fact that this was my first technical interview was really getting to me. Thinking about solving this now, it seems so easy. But at the time I was on a machine I wasn’t used to and essentially performing for someone.
Second Interview
The next problem was rather insane. I think that the second interviewer (who happened to be my friend), went a little crazy selecting this problem. I did learn new things about bit shifting, which was great. But I do believe that the difficulty level of this problem probably cost me the job.
Problem 2
The task for this problem was to take a value as input, and return the value as the binary representation (up to one byte) in reverse. For example, if the number 1 is input, the binary would be 0000 0001
, and the program would return 1000 0000
. I had never tried to do a problem like this before, and the interviewer lead me down a path that was completely new to me. The result was requiring quite a bit more help than the last problem, and probably just following his solution for the problem.
def reverse(value):
result = 0
for i in range(8):
if value & 1 << i:
result += 1 << 7 - i
return result
Its interesting now that I’m writing this out how few lines of code it takes up. It took me probably an hour to come up with this solution. The program works like this:
- Initializes result as 0
- Iterates between 0 and 7
- Uses bit shift to shift a 1 into the place we want to check
- Check if there is a 1 in that position using the bitwise and operator
- If there is a 1, place it that far from the back of the result
- Return the result
Its interesting how unprepared I was for this. I had done work with logical operators and also with bitwise operators, but never bit shifting. I feel as though this is an intense question to ask a new grad who is signing up to write some react code for you!
Conclusion
Going into this interview I practiced quite a few leetcode problems. During the interview I realized that solving leetcode problems at home and solving them in front of somebody are two completely different experiences. I think that if I had dedicated many many hours to leetcode, I may have come across these problems and been more prepared. Going forward I’ll have to study quite a bit more.
This whole process has me thinking about what a strange profession computer science is. For instance, my wife is a biomedical engineer. She has a degree in biomedical engineering and has worked many jobs in her field for a little over 10 years. Never once on an interview was she given any kind of problem to solve. I don’t think you’d really be able to make biomedical engineering problems. Therefore we’re in quite a unique field where potential employers can assess parts of our skillset before we ever do any work for them. I understand the value of solving these kinds of problems, and I’m sure that people who are good at solving these problems are talented software engineers. It does however feel that, at this point, completed projects better represent my overall ability than knowing how to bitshift.
This was an excellent learning experience, and I’m so glad I had the opportunity to experience a technical interview. I think I learned more from this interview than I would have if everything had gone perfectly.
Leave a Reply