I wanted to make adding an entry feel a little bit more responsive. An easy way to accomplish this was to add an alert message when you successfully add to the table. I did this by passing an alert when rendering the template. Alert=0 means an entry was successfully added, and alert=1 would tell them to try again.


This leads me to how I handle duplicate entries. I get the documents in the firebase collection and check each item before adding.
Now, the updating of entries. My thought process was it would be a modal that shows the details of each entry already populated in the fields. The application model has a couple more entries to add so I would need to update the way we implemented that.
So, I created a detail modal and form template. This way I do not clutter one HTML file. I basically took a lot from the previous form that was used to create an entry and added the ‘value’ parameter.
I changed my model classes so that now it is cleaner and will only take the form data to return the object:
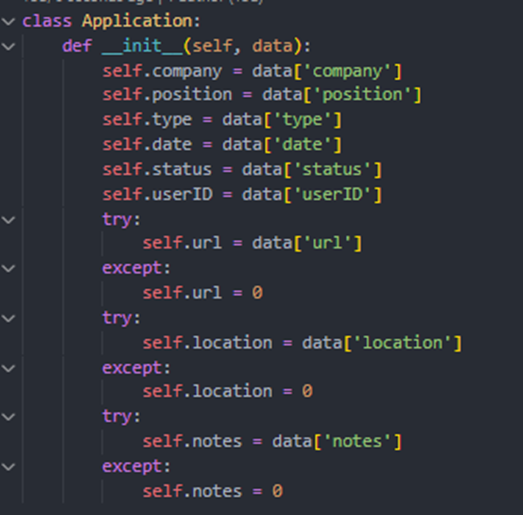
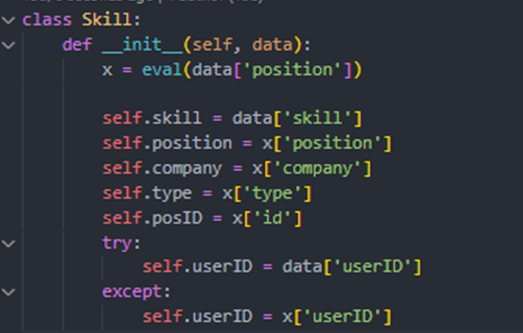
When adding an entry to the database, I made it so that the userID is associated with it. This way my function for getting all the entry documents will check if the userID matches the entry before adding it to the list. Now each user will only see what they added.
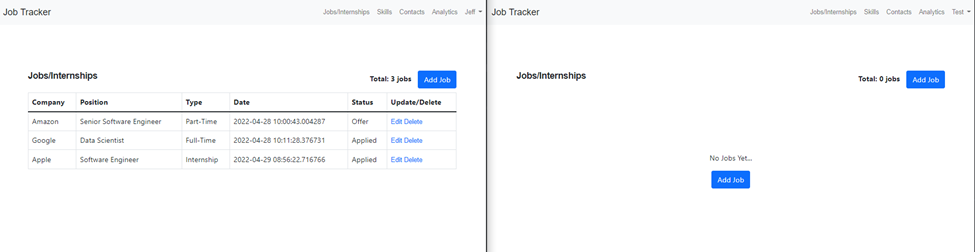
The update functions were pretty straightforward for the skills page. For the jobs page, I had to add extra logic that will also update the skills associated with that job. If you change the name of a company on the jobs page, the table on the skills page should also be changed. This was done by looping through the skills and verifying the IDs.
Running through the functionality I found I was missing something. When I delete a job, the skills associated with that job should also be deleted. I fixed this by also looping through the skills when I am deleting a job and if the IDs match, it also will be deleted.
Overall, this week was a busy one. I got stuck many times. I feel my implementation is not the best and can be improved. Yes, the functionality is working but there may be bugs I am unaware of. This is why having a team is great because there will be more eyes on this, which means they can find what I am missing. This website is starting to take shape!